使用 MediaQuery 和 LayoutBuilders 在 Flutter 中创建手机/平板电脑布局
- 发表于
- flutter
本文将向您展示如何创建根据用户设备(手机或平板电脑)运行的布局。在这里,我们将创建两个 GridView,一个用于手机,另一个用于平板电脑。
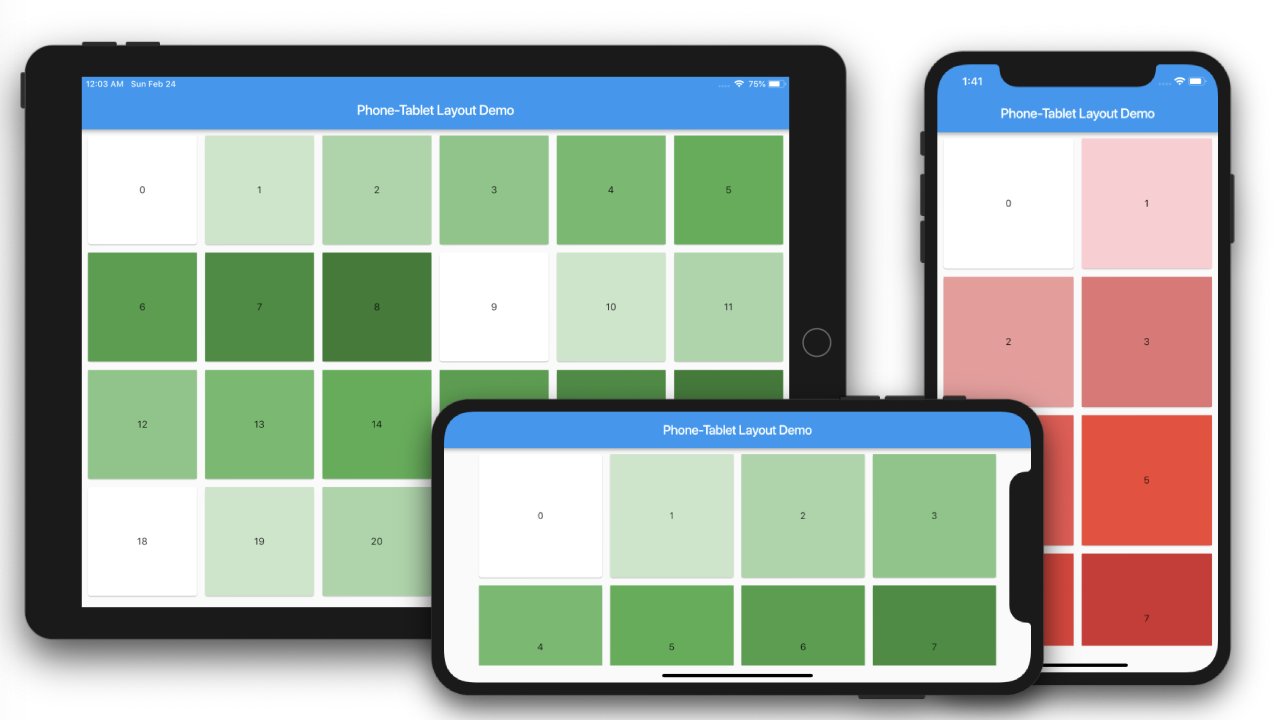
让我们先创建返回两个 GridView 的函数。
gridviewForPhone() {
return Padding(
padding: EdgeInsets.all(5.0),
child: GridView.count(
crossAxisCount: 2,
childAspectRatio: 1.0,
mainAxisSpacing: 4.0,
crossAxisSpacing: 4.0,
children: List.generate(100, (index) {
return Card(
child: Container(
alignment: Alignment.center,
color: Colors.red[100 * (index % 9)],
child: Text('$index'),
),
);
}),
),
);
}
gridviewForTablet() {
return Padding(
padding: EdgeInsets.all(5.0),
child: GridView.count(
crossAxisCount: 4,
childAspectRatio: 1.0,
mainAxisSpacing: 4.0,
crossAxisSpacing: 4.0,
children: List.generate(100, (index) {
return Card(
child: Container(
alignment: Alignment.center,
color: Colors.green[100 * (index % 9)],
child: Text('$index'),
),
);
}),
),
);
}
在这里,Phone GridView 将每行显示 2 个单元格,而平板电脑将每行显示 4 个。
使用 MediaQuery
首先,我们使用 MediaQuery 来确定布局。为此,我们将声明三个变量。
我们假设 600.0 作为平板电脑的边界。
final double shortestSide = MediaQuery.of(context).size.shortestSide; // get the shortest side of device
final bool useMobileLayout = shortestSide < 600.0; // check for tablet
final Orientation orientation = MediaQuery.of(context).orientation; // get the orientation
现在我们的构建方法将更改为
@override
Widget build(BuildContext context) {
final double shortestSide = MediaQuery.of(context).size.shortestSide; // get the shortest side of device
final bool useMobileLayout = shortestSide < 600.0; // check for tablet
final Orientation orientation = MediaQuery.of(context).orientation; // get the orientation
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: useMobileLayout
? gridviewForPhone(orientation)
: gridviewForTablet(orientation),
...
现在我们将方法更改为
gridviewForPhone(Orientation orientation) {
...
GridView.count(
crossAxisCount: orientation.portrait ? 2 : 4
...
}
gridviewForTablet(Orientation orientation) {
...
GridView.count(
crossAxisCount: orientation.portrait ? 4 : 6
}
使用 LayoutBuilder
这是进行比较的示例
LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
if (constraints.maxWidth < 600.0) {
return gridviewForPhone();
} else {
return gridviewForTablet();
}
},
为了简单起见,我们只是使用构建器约束检查设备的最大宽度。
因此,一旦您的设备超过 600.0 的最大宽度,可能在横向模式下它最终会显示平板电脑的 GridView。
完整代码。
原文连接:使用 MediaQuery 和 LayoutBuilders 在 Flutter 中创建手机/平板电脑布局
所有媒体,可在保留署名、
原文连接
的情况下转载,若非则不得使用我方内容。